Enzo Innocenzi created a Laravel Notification channel for the Bluesky social media app, and you can find it on Github. We've been using it for a while now to auto-publish new articles on the site over to our Laravel News Bluesky account. If you are new to Bluesky and want to try it out, Justin Jackson created a Laravel list of many active users on the service.
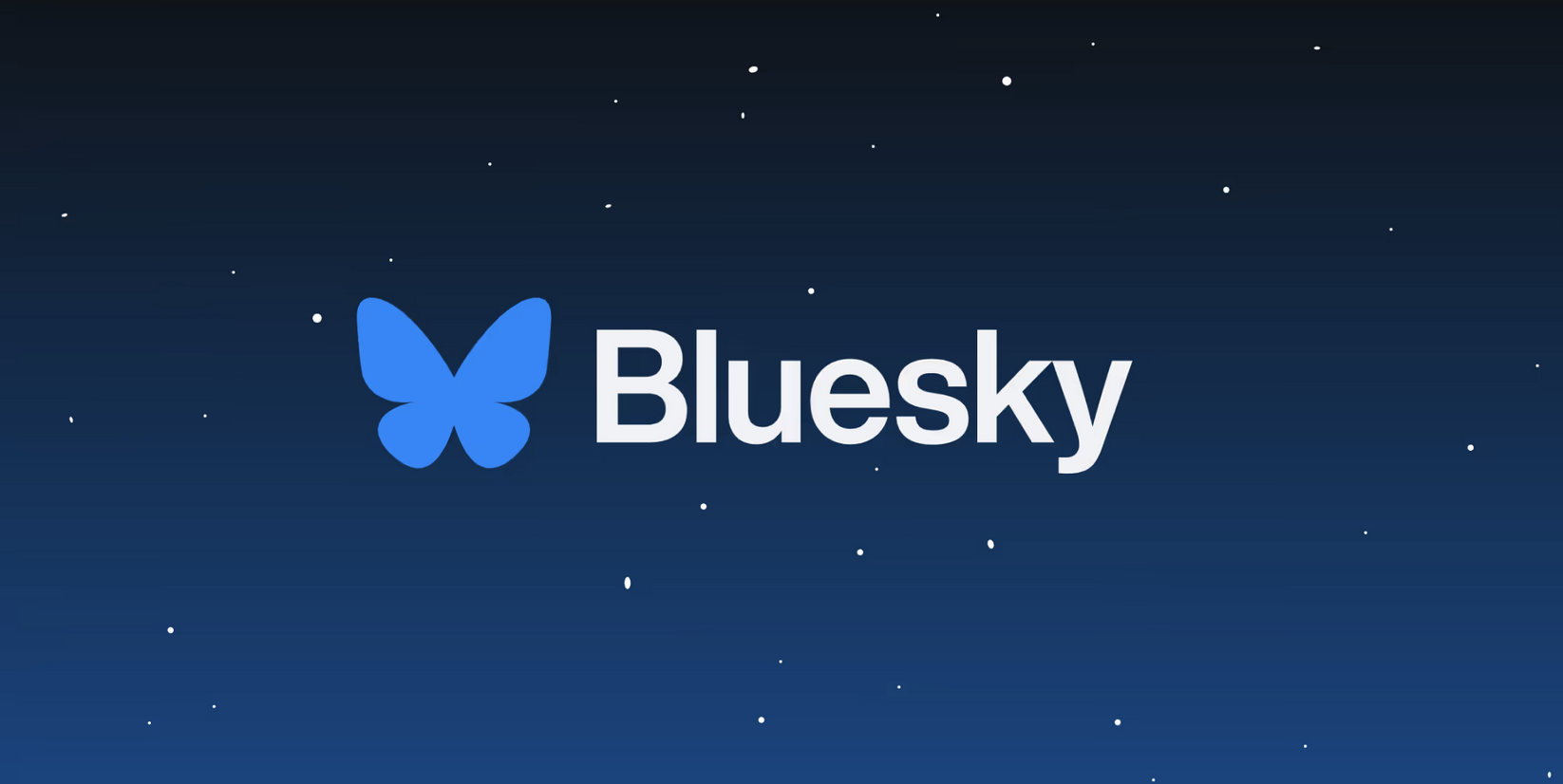
Bluesky notification channel for Laravel
Published on: Oct 30 2024
#Getting started with Bluesky with Laravel
It's super easy to get started, and all you have to do is require the package:
composer require innocenzi/bluesky-notification-channel
Then add this to your .env:
BLUESKY_USERNAME=your-handle BLUESKY_PASSWORD=your-app-password
Then in your config/services.php file:
return [ // ... 'bluesky' => [ 'username' => env('BLUESKY_USERNAME'), 'password' => env('BLUESKY_PASSWORD'), ] ];
Finally, when Laravel Notification:
final class CreateBlueskyPost extends Notification { public function via(object $notifiable): array { return [ BlueskyChannel::class ]; } public function toBluesky(object $notifiable): BlueskyPost { return BlueskyPost::make() ->text('Test from Laravel'); } }
#Laravel News Setup
Here is how ours is set up:
class ArticlePublishedNotification extends Notification { public function via($notifiable): array { return [ BlueskyChannel::class, ]; } public function toBluesky($post): BlueskyPost { return BlueskyPost::make() ->text($post->routes['title']) ->language(['en-US']) ->withoutAutomaticEmbeds() ->embed(new External( uri: 'https://laravel-news.com/'.$post->routes['uri'], title: $post->routes['title'], description: $post->routes['description'], )); }
Find out more about this package on the Github repo and it has full installation instructions and details on how Bluesky handles embeds.